Sidebar
The Sidebar refers to the right menu that you can find in the room, this is generally a good place to communicate with the attendees of an event.
For instance at Livestorm we use this area to put the native Chat, Polls and Questions.
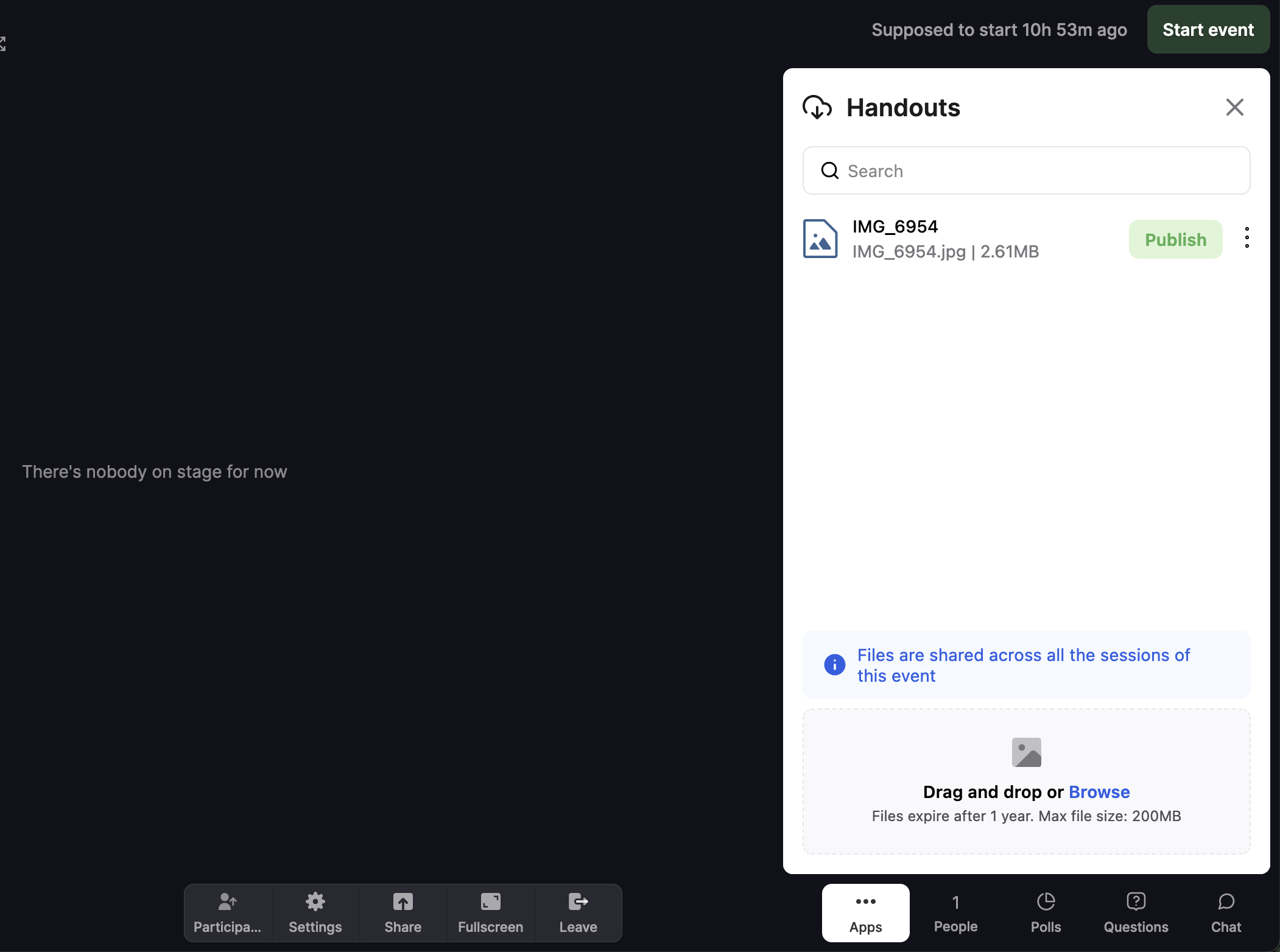
registerPanel
This allows you to declare your Sidebar application.
Calling this method will add your app to the "Apps" menu of the room.
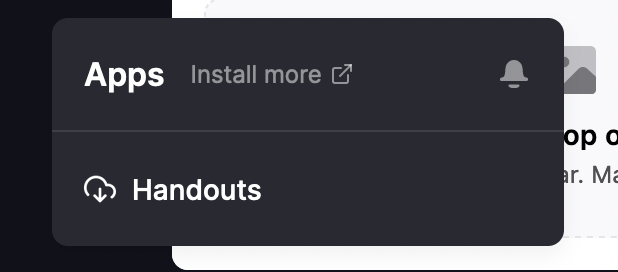
Once a person clicks on your app in the menu it will activate the template
property.
use cases : chat, survey, file sharing, polls, questions
usage :
const app = await Sidebar.registerPanel({
label: 'Handouts',
imageSource: 'https://url/logo.png',
darkImageSource: 'logo.png',
slug: 'handouts',
template: '<p>{{ content }}</p>',
variables: { content: 'hello' },
onMessage: (message) => {
// Do something when using `postMessage({ foo: 'bar' })` from within the template
},
onClose: () => {
// Do something when the sidebar panel is closed
},
onMinimize: () => {
// Do something when the sidebar panel is minimized
},
})
app.focus()
app.setNotificationCount(1)
app.clearNotificationCount()
app.remove()
app.sendMessage({ foo: 'bar' }) // The message can be caught in the template using `window.addEventListener('message', () => {})`
Param | Type | Description |
---|---|---|
label | string | The name of your sidebar app, will show in the menu |
slug | string | The slug that will be used in the URL when the sidebar panel is opened |
imageSource | string | The logo of your sidebar app |
darkImageSource | string | The light version of your logo, will be displayed in the menu which has dark background. Defaults to imageSource if not specified |
template | string | The HTML content you want to display in the modal (can contain CSS or JS) |
variables | Hash | Hash of variables you can interpolate into the HTML template |
onMessage | Function | Function called whenever the postMessage({}) function is called within the HTML |
onClose | Function | Function called whenever the sidebar panel is closed |
onMinimize | Function | Function called whenever the sidebar panel is minimized |
Returns |
---|
Promise< SidebarApp> |
Types
SidebarApp
Method | Params | Description |
---|---|---|
focus | void | Focus your app |
sendMessage | Object | Sends a message to the app's template, can be caught using window.addEventListener('message', () => {}) |
remove | void | Remove the app from the sidebar |
setNotificationCount | number | Display a related notification badge in apps menu |
clearNotificationCount | void | Remove the related notification badge in apps menu |
Updated 7 months ago