OAuth2 authentication
OAuth2 authentication is only available to official technology partners that build public integrations with Livestorm. → Join the program here.
Livestorm's OAuth2 authentication scheme is available to all the official technology partners that want to build an integration with Livestorm on behalf of a Livestorm user. This flow makes it easy for apps developers to connect users with their Livestorm account and automate processes (e.g retrieve engagement data from Livestorm, manage events, registrations, etc.).
This guide is about giving you all the tools required to build a successful and secure OAuth2-based integration with Livestorm.
Requirements
Here are the requirements that you'll need to build an integration with Livestorm through OAuth2:
- Being familiar with the OAuth2 dance. Here's the official OAuth2 spec.
- A secure OAuth2 client which will interact with Livestorm. Your client ID and client secret should be stored securely in your codebase/database, and each individual OAuth2 token should also be stored securely and should never be shared with anyone.
- You'll need a valid OAuth2 redirect URL, served under HTTPS, where users are redirected after a successfully OAuth2 dance with Livestorm. We recommend using ngrok for local purposes so that you can expose your local OAuth2 client to a valid HTTPS URL (otherwise, you can set up a self-serve SSL certificate to serve your local app under HTTPS). This can be useful to expose a temporary, development-only redirect URL endpoint.
- You’ll need to choose the scopes of your OAuth2 app. See the scopes appendix below.
- Finally, you'll need to provide to Livestorm a well-defined (at least 500x500px), square logo with a transparent background.
After providing your app’s scopes, your HTTPS redirect URL, and your logo, Livestorm will give you back a client_id
and a client_secret
. Store them securely in your codebase as they'll allow your app to authenticate your users with their Livestorm account.
OAuth2 flow
The below schema describes how the OAuth2 dance works:
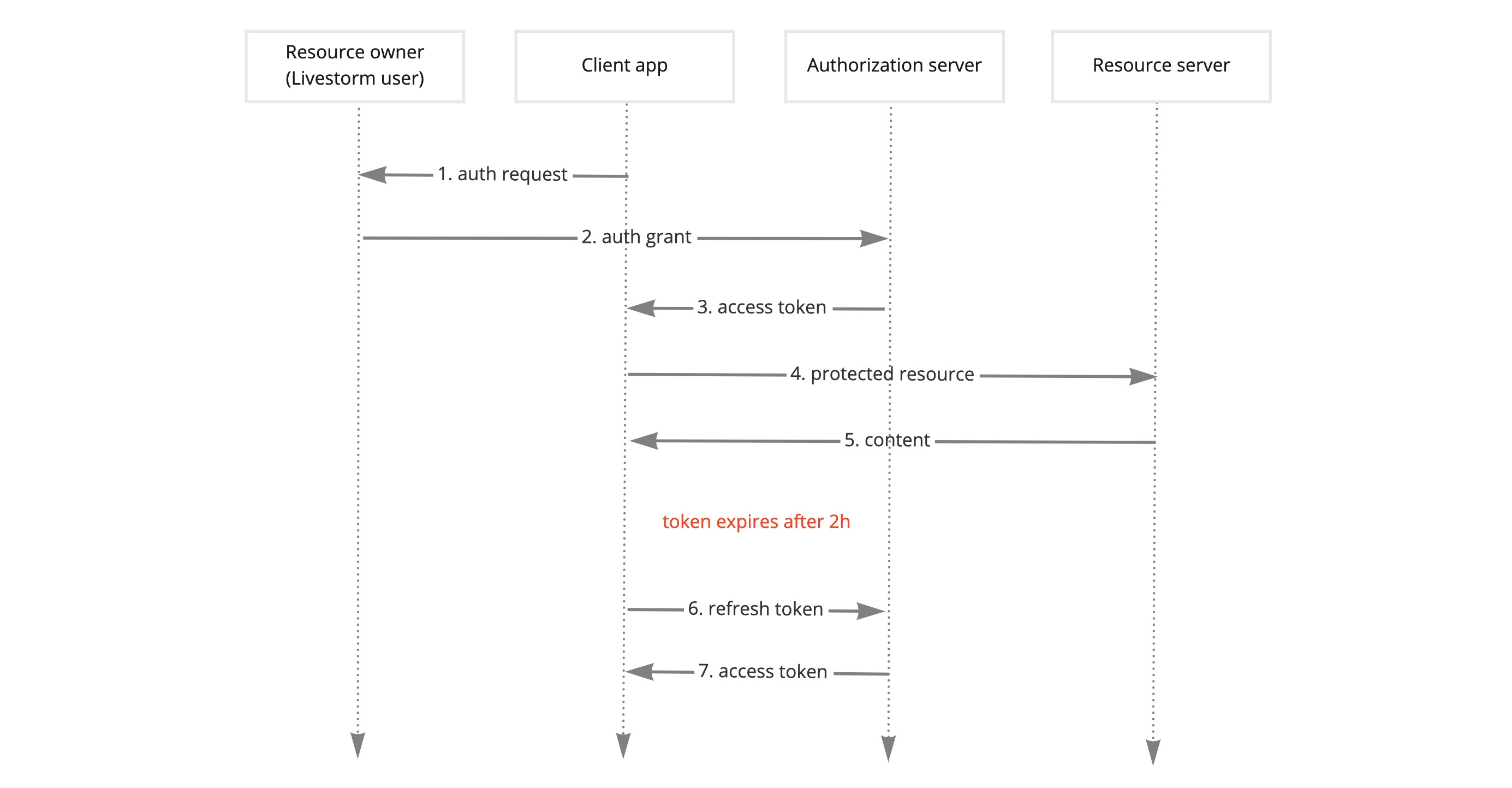
OAuth2 implementation schema
OAuth2 API endpoints
1. Initiate the OAuth2 dance
GET
https://app.livestorm.co/oauth/authorize?response_type=code&client_id=CLIENT_ID&redirect_uri=REDIRECT_URL&scope=SCOPE&state=STATE&code_challenge_method=CHALLENGE_METHOD&code_challenge=CHALLENGE
Replace the above URL with the following variables:
- Replace the CLIENT_ID variable with the
client_id
provided by Livestorm for your app. - Replace the REDIRECT_URL variable with your own redirect URL (it should be the same as the one given to Livestorm when configuring your app). Don't forget to encode your redirect URL.
- Replace the SCOPE section with your space-separated list of scopes (for instance
identity:read events:write admin:read webhooks:write
). As the scopes contain special chars, don’t forget to encode your URL when redirecting users to authenticate:identity%3Aread%20events%3Awrite%20admin%3Aread%20webhooks%3Awrite
. Should a mismatch between the app’s configured scopes and the provided scopes happen, the end-user will see an error when authenticating her Livestorm account. See the details of the available scopes below. - Our authorization server also accepts the STATE, CODE_CHALLENGE, and CODE_CHALLENGE_METHOD parameters from the OAuth2 implementation spec, that your OAuth2 client can provide. The
state
parameter is used to prevent CSRF attacks, and thecode_challenge
andcode_challenge_method
parameters can be used to prevent authorization code interception attacks with the PKCE protection.
Once you built the OAuth2 authorization URL, redirect your user to its location, so that she can give you app access to her Livestorm account. Here’s an example of the authorization page the user will be prompted to accept:
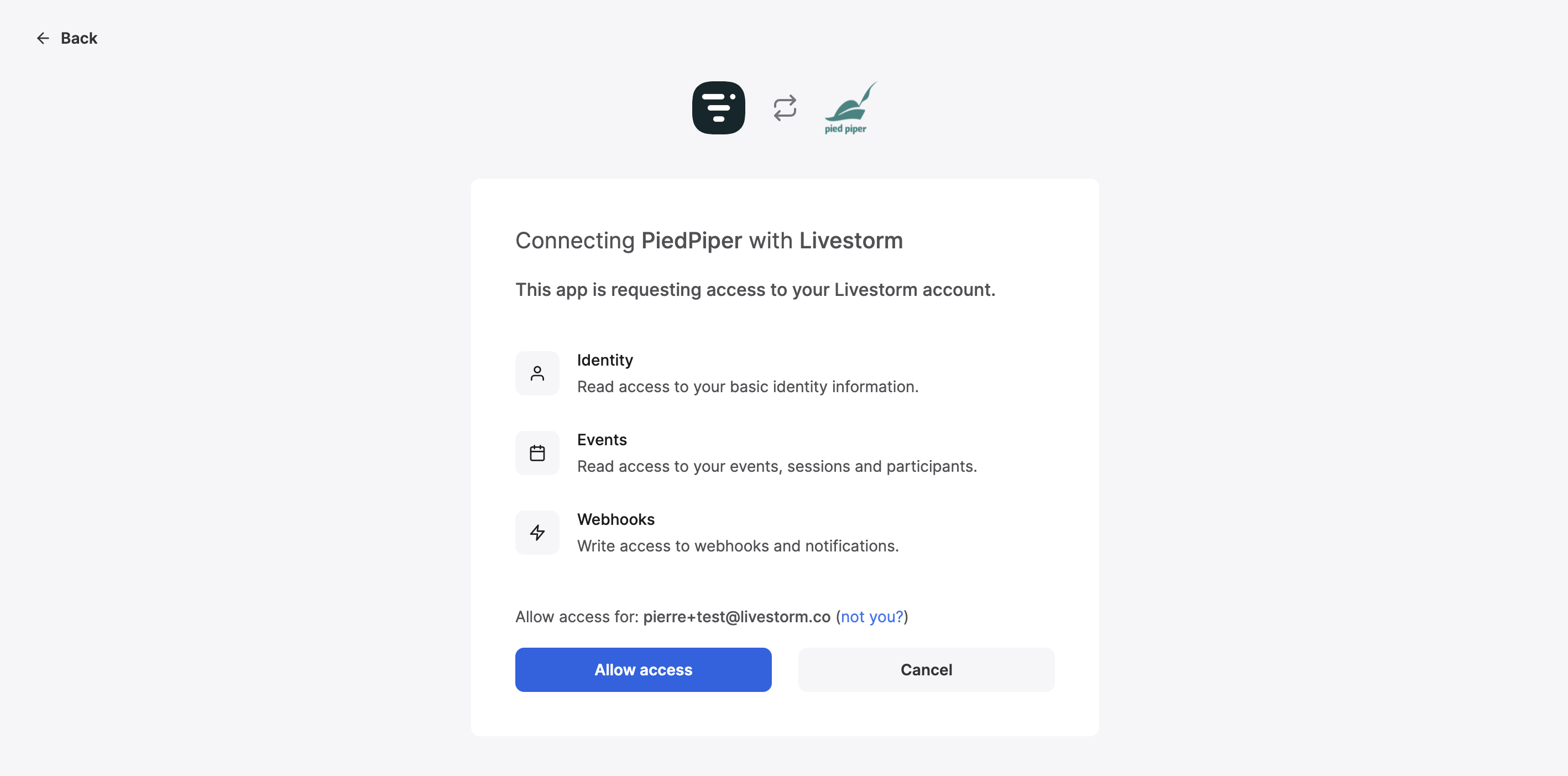
OAuth2 dance end-user validation step
2. Livestorm redirects the user to your app with the authorization code
If the user successfully authenticates her Livestorm account, she'll be redirected back to your redirect URL, with a code
string parameter and the state
that was provided by your server:
/your_redirect_url?code=AUTHORIZATION_CODE&state=STATE
3. Get your access token
Now, you can exchange your authorization code to an access token and a refresh token, that will give you API access to the Livestorm account of this user.
Request
POST
https://app.livestorm.co/oauth/token?grant_type=authorization_code&client_id=CLIENT_ID&client_secret=CLIENT_SECRET&code=AUTHORIZATION_CODE&redirect_uri=REDIRECT_URL
Simply replace this URL with your CLIENT_ID
, CLIENT_SECRET
, REDIRECT_URL
, and the AUTHORIZATION_CODE
that you just received from the previous step.
Here's the response that you should get back from the authorization server:
Response
{
"access_token": "ACCESS_TOKEN",
"token_type": "Bearer",
"expires_in": 7200,
"refresh_token": "REFRESH_TOKEN",
"scope": "SCOPES",
"created_at": 1629205920
}
Please note that the provided access token will expire after 7200 seconds (=2 hours). After that, your access token will no longer work and you'll have to refresh itas explained in step #5.
4. Use the access token to call the API
Now, you can use the freshly retrieved access token to access the API on behalf of this Livestorm user. To do so, simply use any API endpoint from our public API, and use the following authentication header:
curl --request GET \
--url "https://api.livestorm.co/v1/events" \
--header 'Accept: application/json' \
--header 'Authorization: Bearer ACCESS_TOKEN'
Please note that, as opposed to the regular API tokens, OAuth2 tokens need to be prefixed with the
Bearer
keyword.
5. Refresh your access token
Your OAuth2 access token will expire after 2 hours. All the information will be sent in the JSON response of the POST /oauth/token endpoint to help you know when the user's access token will expire. In order to refresh this token with a new one, use the following endpoint:
POST
https://app.livestorm.co/oauth/token?grant_type=refresh_token&client_id=CLIENT_ID&client_secret=CLIENT_SECRET&refresh_token=REFRESH_TOKEN
Simply replace the above URL with your own CLIENT_ID
, CLIENT_SECRET
, and the user's current REFRESH_TOKEN
. The response will be equivalent to the one received in step #3.
OAuth2 apps’ API rate limits
Validated partners will be provided a single burst rate API limit of 5 API requests per second per user access token.
OAuth2 scopes
For security reasons, your OAuth2 app needs to be configured with a specific set of scopes. Scopes ensure that an app only requests access to required data for end-users. By default, apps should follow the principle of data minimization to only require what’s needed (see EU’s GDPR guidelines on this topic).
Livestorm provides apps developers a list of scopes that can be chosen (and sometimes combined) to fullfil a job to be done:
full:read
: This scope gives full read-only access to all API endpoints to the currently connected user. It can only be combined with other individual write scopes.full:write
: This scope gives full read/write access to all endpoints on behalf of the currently connected user. It cannot be combined with any other scopes.identity:read
: This scope gives read-only access to identity-related endpoints (e.g the /me and /organization endpoints).identity:write
: This scope gives full read/write access to identity-related endpoints (e.g the /me and /organization endpoints).events:read
: This scope gives read-only access to all endpoints related to events, sessions, and participants (e.g the /events[/*], /sessions[/*], and /people/[/*] endpoints).events:write
: This scope gives read/write access to all endpoints related to events, sessions, and participants (e.g the /events[/*], /sessions[/*], and /people/[/*] endpoints).admin:read
: This scope gives read-only access to all endpoints related to account administration (e.g the /users[/*] and /organization endpoints).admin:write
: This scope gives read/write access to all endpoints related to account administration (e.g the /users[/*] and /organization endpoints).webhooks:read
: This scope gives read-only access to all webhooks-related endpoints (e.g /webhooks[/*]).webhooks:write
: This scope gives read/write access to all webhooks-related endpoints (e.g /webhooks[/*]).
The full:read
scope includes read-only access to all xxx:read
sub-scopes, and the full:write
scope gives access to all other scopes. Both of these scopes require strict scrutiny as they’re quite permissive for end-users.
Submit an OAuth2 request
Would you like to integrate your own app with Livestorm and be listed in our marketplace as an official partner?
Updated 5 months ago