Chat
broadcast()
Broadcasts a message in the chat.
Message will be displayed to all the recipients of the chat
use cases : sending custom media (video, audio, GIFs), polls, call to actions
usage :
Chat.broadcast({
text: 'Hello world',
html: '<p>Hi</p>'
})
The
html
property uses the template system. We recommend you to use our UI Kit to build your HTML templates.
Param | Type | Description |
---|---|---|
text? | String | The text of the message |
html? | String | An HTML template that will be rendered below the text |
@returns
An instance of the created Message that you can use to delete the message or be notified whenever the HTML posts message
send()
Adds a message in the chat.
Message will be sent locally, only to the connected user
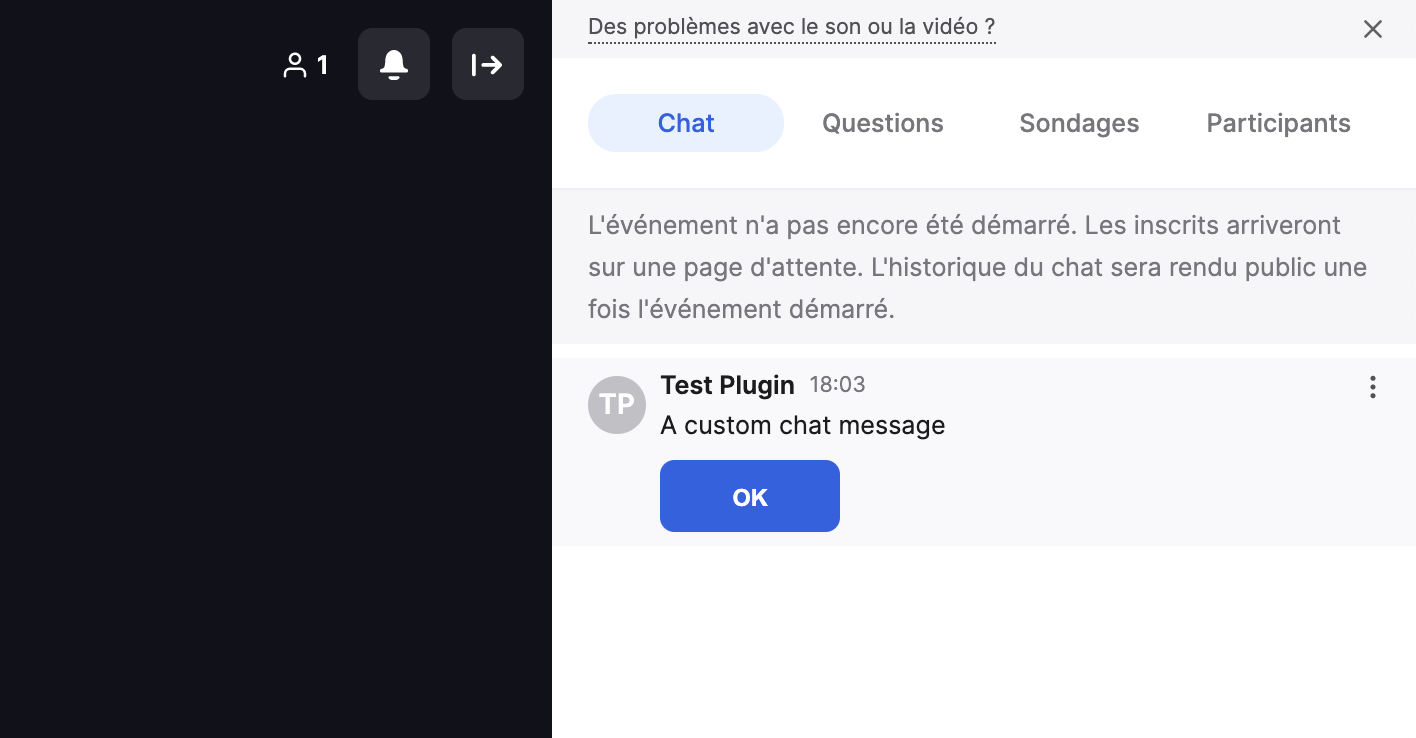
use cases : sending user specific content (video, audio, GIFs), polls, call to actions, forms
usage :
Chat.send({
user: {
firstName: 'Michael'
},
text: 'Hello world',
html: '<p>Hi</p>'
})
The
html
property uses the template system. We recommend you to use our UI Kit to build your HTML templates.
Param | Type | Description |
---|---|---|
user | Hash | A hash with informations about the sender (firstName, lastName, avatarUrl) |
text | String | The text of the message |
html | String | An HTML template that will be rendered below the text |
@returns
An instance of the created Message that you can be used to delete the message or be notified whenever the HTML posts message
listen()
Be notified whenever the current user or all users post messages in the chat.
use cases : chat bots, forwarding messages to another API (slack, intercom), language filter, translations, notifying moderators of specific things
usage :
Chat.listen(message => {
console.log(`Someone said ${message}`)
}, { everyone: true })
Param | Type | Description |
---|---|---|
callback | Function(message) | Function that will be called whenever the user posts a message |
options | { everyone?: boolean } | If everyone is true messages coming from other users will also be caught |
intercept()
Intercept Chat messages matching a specific regex.
Once intercepted, the message will not be displayed in the chat.
use cases : custom chat commands, trigger actions, chat bots, forwarding messages to another API (slack, intercom), language filter, translations, notifying moderators of specific things
usage :
Chat.intercept(/lol/, (message) => console.log(`someone said ${message}`))
Param | Type | Description |
---|---|---|
matcher | RegExp | A regular expression that represents the messages to be intercepted |
callback | Function(message) | A function that will be called whenever a message that matches your criteria is intercepted |
registerCommand()
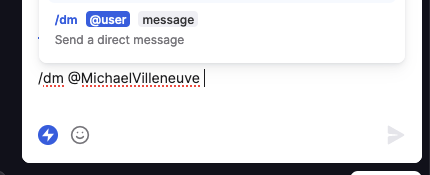
Register a chat command in the commands menu. This API is a great way to provide quick actions and shortcuts for team members willing to boost their productivity and can be used to execute various actions involving the chat (sharing custom content, files, videos, etc).
Chat.registerCommand({
command: 'dm',
label: 'Send a message',
params: ['name', 'message']
onTrigger: ({ params }) => {
console.log(`${params.name} said ${params.message}`
}
})
Param | Type | Description |
---|---|---|
command | String | The command name, users will type "/" followed by the value of this param |
label | String | The description of your command |
params | Array<String> | The list of parameters that your command will receive |
onTrigger | Function<ChatCommandTrigger> | Function called whenever a user types your command even if params are missing |
Types
ChatCommandTrigger
Param | Type | Description |
---|---|---|
params | Object | An object containing one key per param sent to the command |
variables | Hash | Hash of variables you can interpolate into the HTML template |
rawCommand | String | The raw message content, including command name |
valid | Boolean | Whether all parameters have been filled |
mentions | Array<{ replacedWith: string, user: User }> | List of users that have been mentioned using the @name syntax |
scope | String | The scope in which your message has been sent |
registerMessageAction()
Register an entry in the context menu of a chat message.
Can be used to trigger any action (sharing custom content, files, videos, etc)
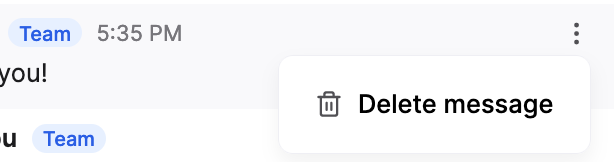
use cases: message translation, actions specific to the user who sent the message
usage:
Chat.registerMessageAction({
label: 'Translate this message',
imageSource: 'http://image/image.png',
onClick: (message) => console.log('someone clicked this button')
})
Param | Type | Description |
---|---|---|
label | string | The label of the action |
icon | string | An icon from the https://feathericons.com library (not recommended) |
imageSource | string | URL of an icon (png, svg) that will be displayed next to your button |
onClick | Function | Function called whenever someone clicks on your button |
Buttons.registerChatShareButton()
Register an entry in the Share menu of the chat.
Can be used to trigger any action (sharing custom content, files, videos, etc)
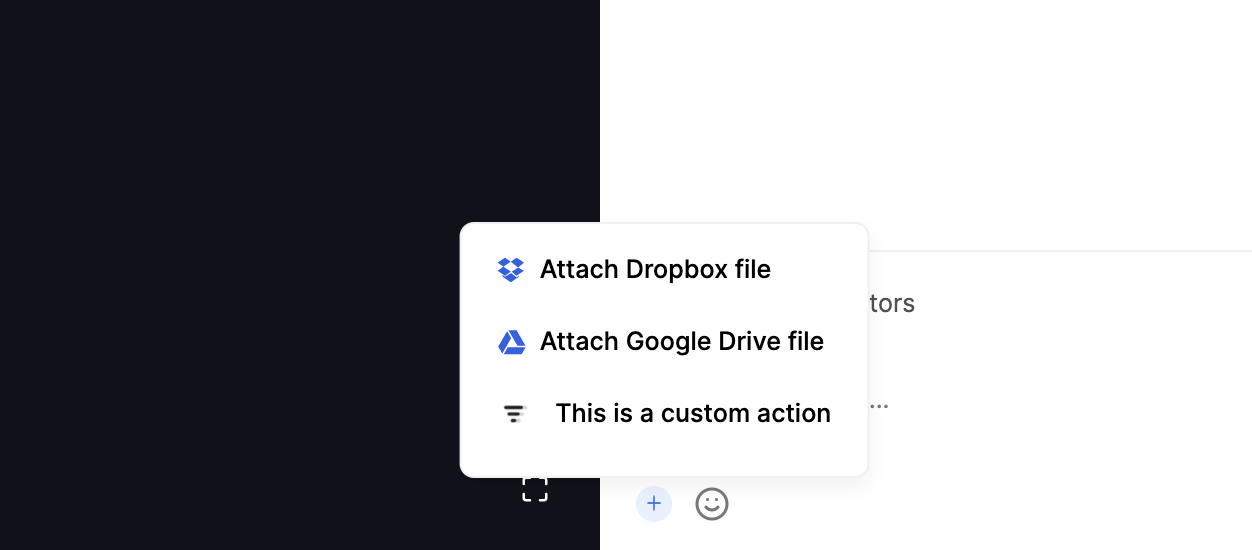
use cases : trigger actions that will affect the chat (sending files, starting workflows, etc)
usage :
Chat.Buttons.registerChatShareButton({
label: 'Share a Document',
imageSource: 'http://image/image.png',
onClick: () => console.log('someone clicked this button')
})
Param | Type | Description |
---|---|---|
label | String | The label of the action |
icon | String | An icon from the icons list |
imageSource | String | URL of an icon (png, svg) that will be displayed next to your button |
onClick | Function | Function called whenever someone clicks on your button |
Updated 3 months ago