Tracking
The Tracking
API is a collection of APIs and Configuration that can be used to display statistics about your plugin usage.
At Livestorm we use it to show detail about the Emoji Reaction plugin usage such as the number of reaction sent per user and which reaction has been sent.
Tracking.Event.track()
Prior to sending events from your plugin's code, you need to configure how your events will be displayed in the dashboard.
Read theConfiguration
section below to know more
Track an event to make it visible in the dashboard.
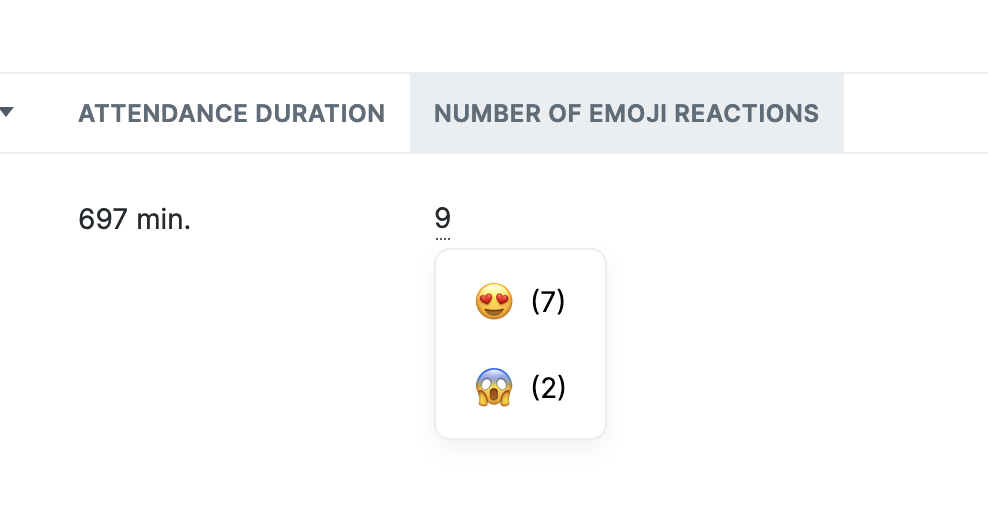
use cases : Displaying usage statistics, provide insights to your team members
usage :
Tracking.Event.track({
key: 'emoji-reactions',
value: 'joy'
})
Param | Type | Description |
---|---|---|
key | String | The key defined in your livestorm.config.js |
value | String | The value to be tracked, it can be omitted if your aggregation is increment (making it a simple counter) |
Configuration
This step is mandatory to have your events displayed in the dashboard. You will need to update your livestorm.config.js
file to leverage the new tracking syntax.
Basically you need to add a tracking
object, which contains an events
array, just like below:
"tracking": {
"events": [
{
"name": {
"en": "Handouts downloads",
"fr": "Nombre de tΓ©lΓ©chargements",
},
"key": "handouts",
"aggregations": ["group_array", "array", "increment"],
}
]
},
Here is a list of parameters your can pass to each event
object
Param | Type | Description |
---|---|---|
name | Object | An object of translations that will be used to display the column name in the dashboard |
key | String | The key to be used with the Tracking.Event.track API |
aggregations | Array<"group_array", "array", "increment", "average", "string"> | The type of aggregation to be displayed |
delete_existing_events | boolean | If your plugin is published with this param, it will automatically reset the event list upon publish |
value_type | boolean, image, url, string, number | Providing this will affect the way the value is displayed in the dashboard and will also add validations to the input value |
A note about the aggregations
Aggregations will affect the way the data is computed on the dashboard.
Despite being a list, as of today only the first element of the aggregation array will be used in the dashboard. In the future team members will be able to use any of the provided aggregation.
increment
increment
This is the simplest aggregation possible, it will simply act as a counter of events.
For instance, the following code
Tracking.Event.track({ key: 'test' })
Tracking.Event.track({ key: 'test' })
Will simply show "2" in the created column in the dashboard.
array
array
The array aggregation will act as a simple listing of the values sent using the tracking API.
Tracking.Event.track({ key: 'test', value: 'apples' })
Tracking.Event.track({ key: 'test', value: 'oranges' })
Will simply display apples, oranges
in the column for the given user.
The mechanism simply takes all values an apply a join(', ')
on the array.
average
average
The average aggregation requires a number to be given as value. Then it will simply compute an average of the given values.
Tracking.Event.track({ key: 'test', value: 10 })
Tracking.Event.track({ key: 'test', value: 5 })
Will show 7.5
in the dashboard column.
string
string
This isn't technically an aggregation, what this does is simply take the latest event for the given user.
Tracking.Event.track({ key: 'name', value: 'Michael' })
Tracking.Event.track({ key: 'name', value: 'John' })
It will simply display John
in the dashboard. This is useful if you want to display the result of something the user can update over time, like a field value.
group_array
group_array
This is currently the most powerful aggregation. It allows users to know the total of events for an event, but also to see the detail of every sent values
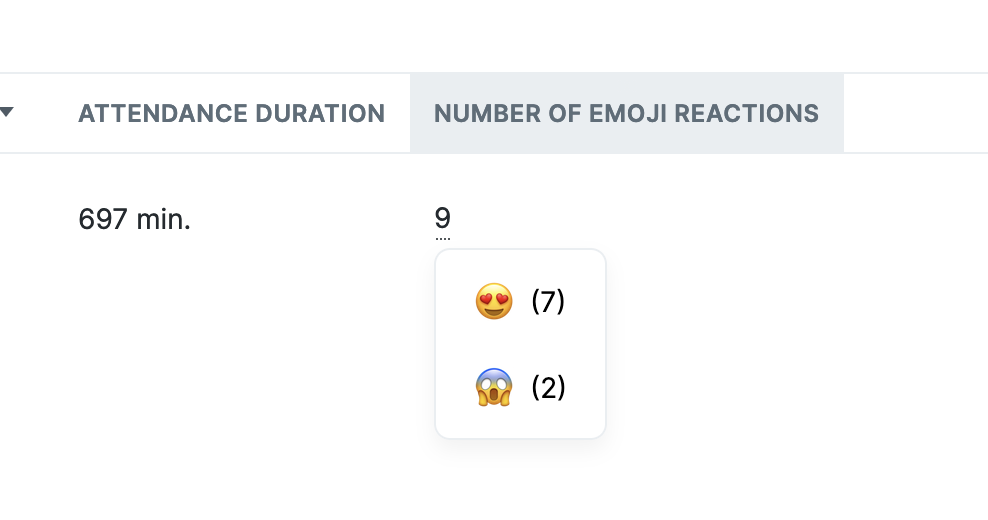
To get the same result as the above image, you'd need to send:
for (let i = 0; i < 7; i++) {
Tracking.Event.track({ key: 'test', value: 'π' })
}
Tracking.Event.track({ key: 'test', value: 'π±' })
Tracking.Event.track({ key: 'test', value: 'π±' })
Updated 8 days ago