Streams
The Streams
API is a collection of tools you can use to create and manage the streams visible inside the room.
Methods
addStream()
addStream()
Add a custom HTML media stream to the stage.
This allows you to display completely custom content to everyone in the room.
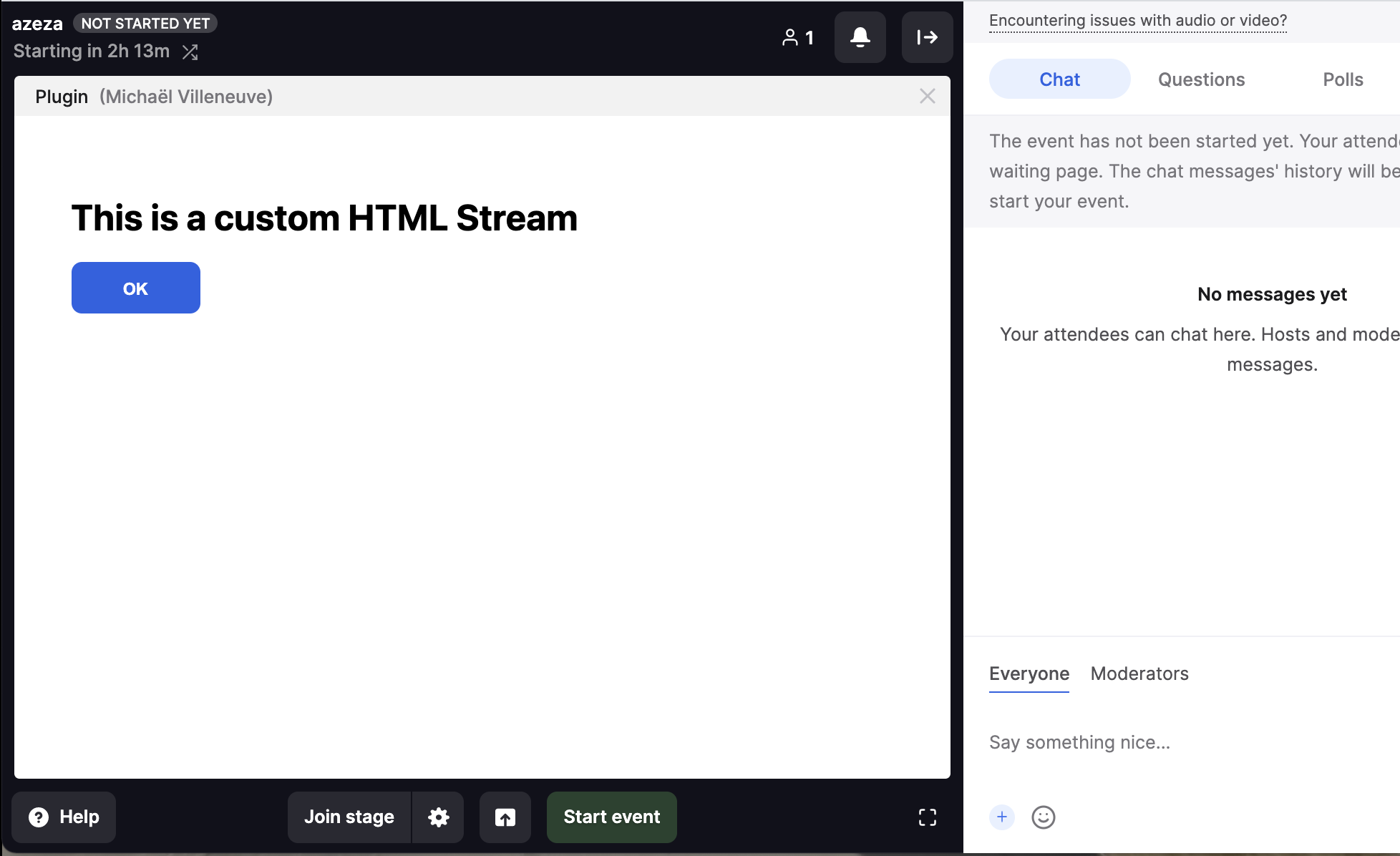
use cases : embed website, embed video, live feed, live collaboration tools, games, etc. It's the best API to provide content that illustrates the subject of the event and that is synced between participants.
usage :
Streams.addStream({
title: 'Stream title',
imageUrl: 'htt://....',
template: '<p>{{ content }}</p>',
variables: { content: 'Live stream' },
onMessage: (message) => console.log('the stream send a message')
})
When your stream gets activated, inside your template, you can listen to the register event like this:
window.addEventListener('message', (message) => {
const event = message.data
if (event.eventName === 'register') {
console.log(event.data.isStreamOwner)
}
});
The register
event contains a data property named isStreamOwner
that allows you to know whether the person who shared the stream is yourself.
Thereby allowing you to activate some custom UI, for instance control buttons for the admin who shared the stream.
To communicate with other attendees streams from within the template you can use the postMessageToEveryone({ })
function that will also trigger the addEventListener
function on everyone's browser.
The
Streams.addStream
API uses the template system. We recommend you to use our UI Kit to build your HTML templates.
You'll find some code examples of this API in our Livestorm Plugins Examples repository.
Params | Type | Description |
---|---|---|
template | String | The HTML template that will be rendered inside the stream |
title | String | Title of the stream |
imageUrl | String | Logo of the stream |
variables | Hash | A hash of variables that you want to interpolate within the document |
onMessage | Function | Function called whenever the stream posts a message |
Returns |
---|
Promise<Stream> |
registerCameraEffect()
registerCameraEffect()
Expose a custom video effect. Gives the ability to the user to select an input stream effect.
This API can be used to create stream with effects such as (background blur, filters, OSD, etc)
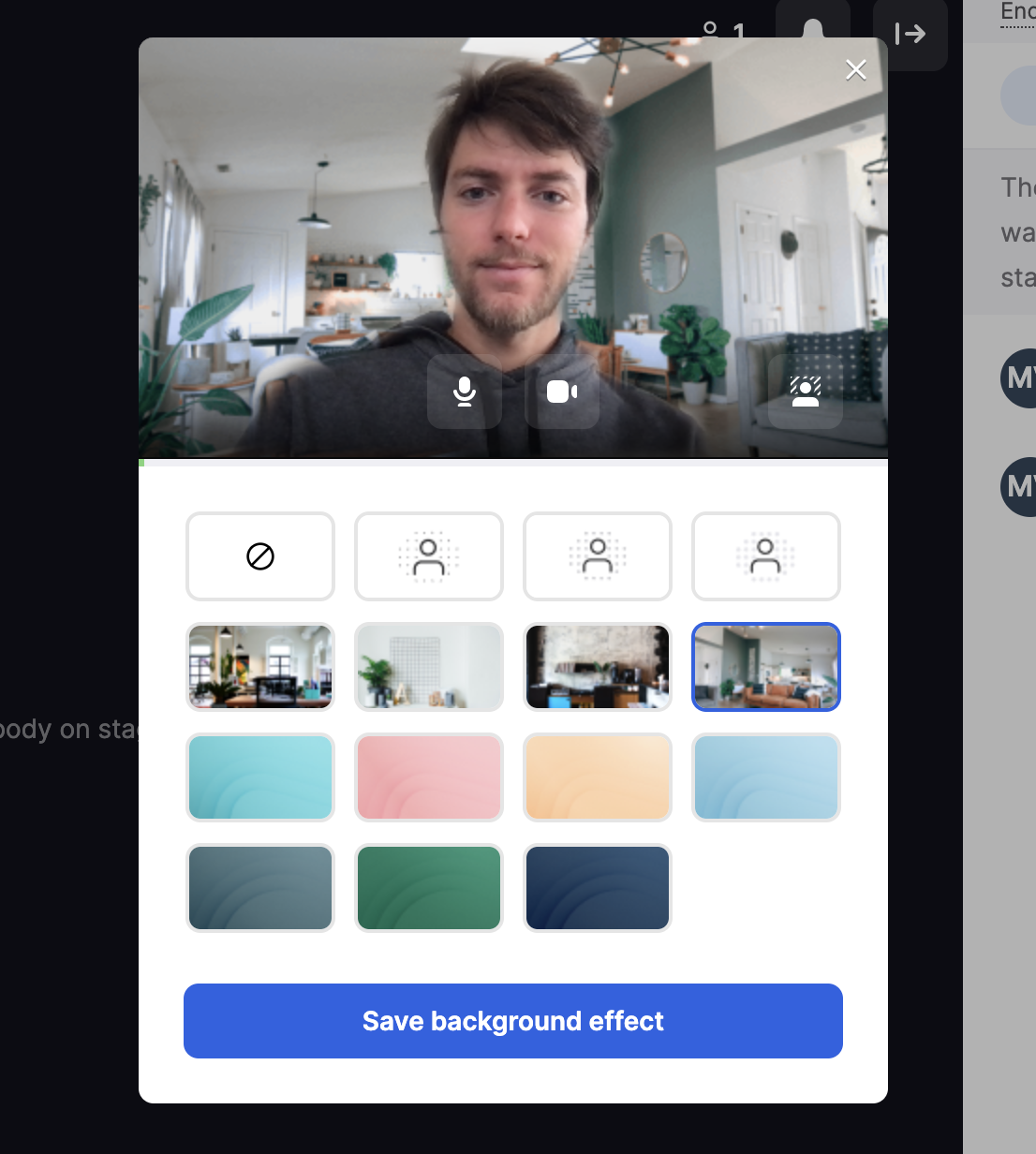
Registering a video effect consists of 2 steps :
- calling the registerCameraEffect function
- modifying the
canvas
variable as you wish from the given HTML template
Inside the iframe you need to declare a function setupStream
. It will be called when the user selects your video effect.
Your setupStream function will be responsible for refreshing the canvas at every frame you wish to send.
The appearance of the canvas will be streamed to other participants using the captureStream function
To add effects on top of the webcam stream, you can use the
video
variable that already contains the user's webcam stream inside a<video>
HTML tag.A way to do so is to simply write the video output to the canvas, and refresh it at 30FPS :
setInterval(() => { canvas.getContext("2d").drawImage(video, 0, 0, canvas.width, canvas.height) }, 1000/30)
You'll find some complete code examples on Github right here
Analyzing and altering video streams can be CPU/GPU demanding, please thoroughly test your camera effects on multiple browsers and devices
Be aware that canvas exposed via this API must not make cross origin calls (fetch, images, etc).
Consider using small images encoded as base64 for instance.
use cases : face filters, virtual backgrounds, gesture recognition, OSD
usage :
const template = `
<script>
function setupStream() {
setInterval(() => {
/* do something with the canvas variable and video variable
}, 1000/30)
}
</script>
`
Streams.registerCameraEffect({
template,
variables: { foo: 'bar' }
})
Params | Type | Description |
---|---|---|
label | String | A label to indicate the purpose of your video effect |
template | String | The HTML document that creates the video stream |
variables | Hash | A hash of variables that you want to interpolate within the document |
Returns |
---|
CameraEffectWrapper |
registerMultipleCameraEffects()
registerMultipleCameraEffects()
Expose multiple effects with a single template.
This allows to get a quicker feedback when selecting a template by switching effect without reloading the entire iframe component.
The internal of this API is similar to registerCameraEffect, please refer to its documentation for a better understanding
Example
Streams.registerMultipleCameraEffects({
template: `<script>window.setupStream = () => publishStream()</script>`,
effects: [
{
variables: { foo: bar },
label: 'Moon',
id: 'Moon'
}
]
})
param | type | description |
---|---|---|
template | String | The HTML document that creates the video stream |
effects | Array<{ variables: {}, label: string, id: string }> | An array of effects |
Returns |
---|
CameraEffectWrapper |
Types
Stream
Stream
Methods | Returns | Description |
---|---|---|
update(data: Object) | void | Update the content of a stream |
destroy() | void | Remove the stream from the stage |
CameraEffectWrapper
CameraEffectWrapper
Methods | Returns | Description |
---|---|---|
sendMessage(data: Object) | void | Send a message to the camera effect |
Updated 11 months ago