Stage
The Stage
API is a collection of APIs that can be used to add interactions and buttons to the stage.
Stage.Buttons.registerShareButton()
Register a new entry in the Share menu dropdown, only available to contributors.
This action can be used to trigger any action (sharing custom content, files, videos, etc).
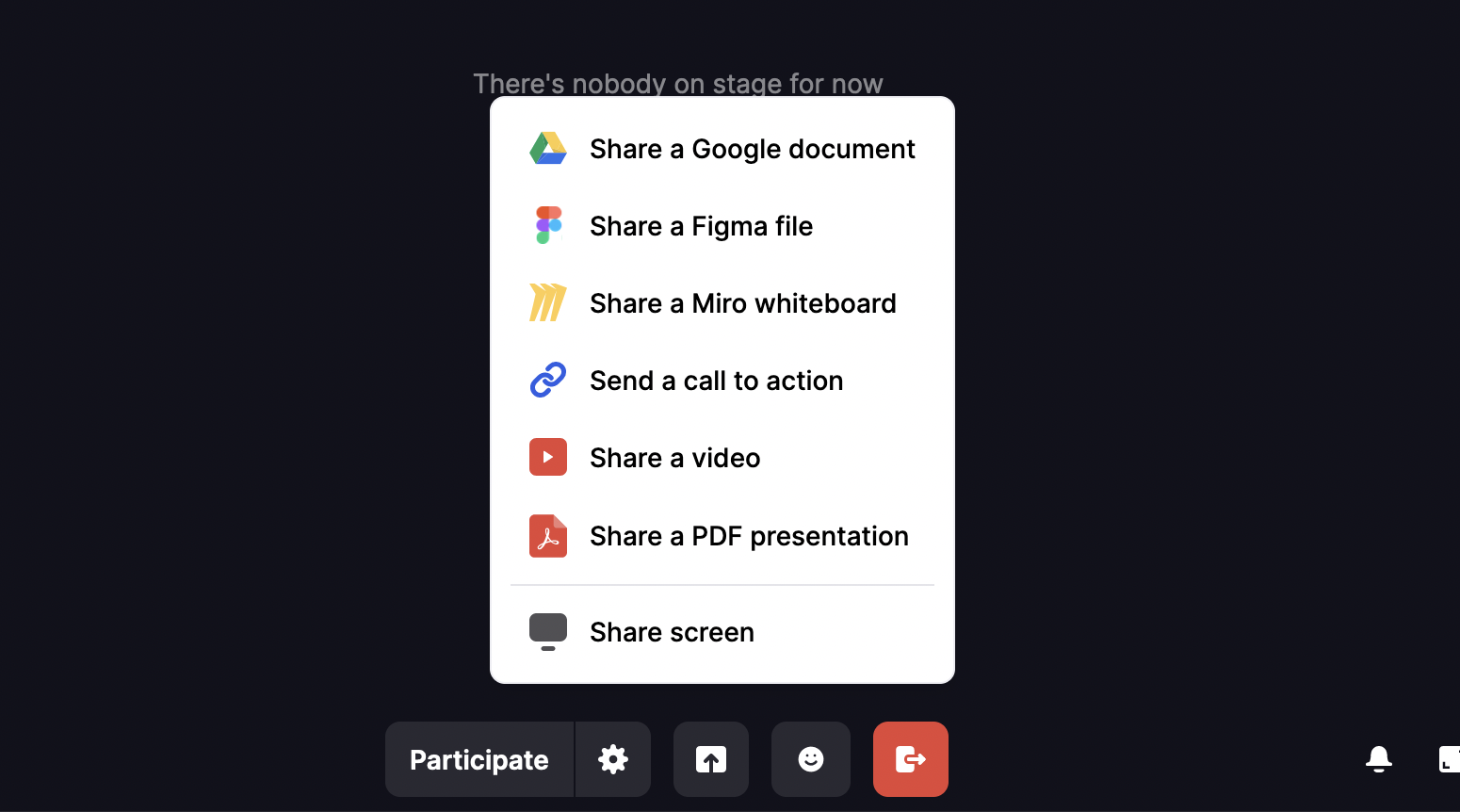
use cases : triggering actions, menus
usage :
Stage.Buttons.registerShareButton({
label: 'Share a Document',
imageSource: 'http://image/image.png',
onClick: () => console.log('someone clicked this button')
})
Param | Type | Description |
---|---|---|
label | String | The label of the button |
icon | String | A Feather icon name |
imageSource | String | A custom icon to be displayed next to your button |
onClick | Function | A callback called when someone clicks on your button |
Note: You should either use the icon
or imageSource
parameters, but not both at the same time! For the latter, don't hesitate to use the livestorm asset command to upload your own logo.
Stage.Buttons.registerStageButton()
Register a new entry in the stage actions (for both contributors and participants).
These actions can be used to trigger any action (sharing custom content, files, videos, etc).
You can use it to show a submenu just like the image below, or as a simple click button that triggers any other subsequent workflow.
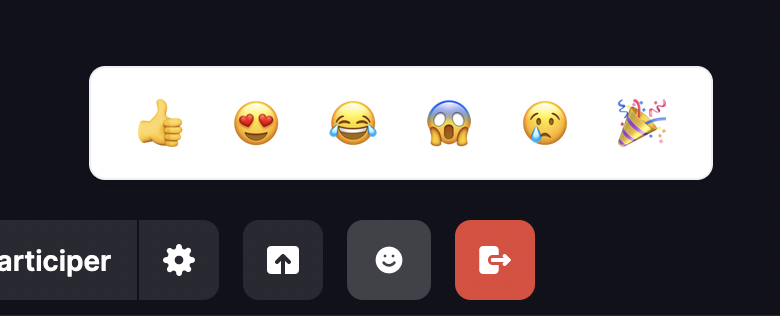
use cases : triggering actions, menus
usage :
// Display the button
const button = Stage.Buttons.registerStageButton({
label: 'React with an emoji',
icon: 'smile',
imageSource: 'https://plugin-asset...',
// Optional: displays a custom menu on top when clicking on the button
iframe: { template, variables, width, height, onMessage },
// Optional: displays a dropdown on top when clicking on the button
dropdownActions: [{ name: '😱', label: '😱' }],
// Not called if iframe option is specified
onClick: (payload) => {}
})
// Remove the button
button.remove()
Note: The dropdownActions
param should not be used at the same time as the iframe
param. Both can be used to display content as a submenu shown when the button is clicked.
Also note that when using the iframe
property the onClick
function will never be called. Instead, you should handle the actions within the iframe
and with the onMessage
property of the iframe
object.
The iframe property uses the template system. We recommend you to use our UI Kit to build your HTML templates.
Param | Type | Description |
---|---|---|
label | String | The label of the button |
imageSource | String | URL of an icon for your button |
darkImageSource | String | URL of a dark icon for your button |
icon | String | A Feather icon name for your button |
iframe | { template, variables, width, height, onMessage } | A frame to be displayed on top of your button whenever clicked on |
dropdownActions | Array<{name, label}> | A list of actions to be displayed on top of your button whenever clicked on |
onClick | Function | A callback called when someone clicks on your button |
Updated 5 months ago